在这篇文章中,我们将深入探讨如何实现一个头像裁剪组件 (AvatarCropper),它不仅可以裁剪图片,还能处理缩放、旋转等操作。该组件基于 Taro 框架构建,可以在多种环境中运行(如微信、支付宝小程序和Web)。我们将分析其核心功能,结构,以及如何使用它。
组件概述
AvatarCropper 是一个用户自定义的头像裁剪工具,允许用户选择图片进行裁剪,支持缩放、旋转、拖动等操作。组件的功能包括:
• 选择图片进行裁剪。
• 支持缩放和旋转图像。
• 在画布上实时显示裁剪效果。
• 裁剪完成后,可以返回裁剪后的图片或文件路径。
组件属性解析
AvatarCropper 的一些常见属性:
• maxZoom:最大缩放倍数,控制裁剪区域的最大缩放程度。
• space:裁剪框与画布边缘的空隙,避免裁剪框被画布边界限制。
• toolbar:裁剪工具栏的内容,可以自定义显示的按钮。
• toolbarPosition:工具栏的位置,支持 top 或 bottom。
• editText:显示在裁剪工具栏的编辑文字。
• sizeType 和 sourceType:允许选择图片的类型和来源。
• shape:裁剪框的形状,支持 square 或 round。
• onConfirm:裁剪完成后的回调函数,传递裁剪后的图片路径。
• onCancel:取消裁剪的回调函数。
代码
index.less code
.avatar-cropper {
position: relative;
display: flex;
&-edit-text {
position: absolute;
top: 0;
left: 0;
width: 100%;
height: 100%;
background-color: #000;
z-index: 1;
color: #fff;
display: flex;
justify-content: center;
align-items: center;
}
&-input {
position: absolute;
top: 0;
left: 0;
width: 100%;
height: 100%;
opacity: 0; // 隐藏原生上传按钮
cursor: pointer;
z-index: 2;
}
&-popup {
position: fixed;
top: 0;
left: 0;
width: 100%;
height: 100%;
background-color: #000;
z-index: 1000;
&-canvas,
&-cut-canvas {
position: absolute;
bottom: 0;
left: 0;
width: 100%;
height: 100%;
z-index: 1;
}
&-cut-canvas {
z-index: 0;
}
&-toolbar {
position: absolute;
bottom: 30px;
left: 0;
width: 100%;
z-index: 2;
&.top {
top: 0;
bottom: inherit;
}
&-flex {
width: 100%;
display: flex;
justify-content: space-between;
}
&-item {
// flex: 1;
color: #fff;
padding: 10px 30px;
cursor: pointer;
display: flex;
align-items: center;
}
}
&-highlight {
position: absolute;
left: 0;
top: 0;
width: 100%;
height: 100%;
z-index: 1;
background-color: transparent;
.highlight {
position: absolute;
left: 50%;
top: 50%;
transform: translate(-50%, -50%);
background-color: transparent;
// box-shadow: 0 0 1000px 1000px rgba(0, 0, 0, 0.6);
}
}
}
&.round {
.nut-avatar-cropper-edit-text {
border-radius: 50%;
}
}
}
[dir='rtl'] .avatar-cropper,
.nut-rtl .avatar-cropper {
&-edit-text {
left: auto;
right: 0;
}
&-input {
left: auto;
right: 0;
}
&-popup {
left: auto;
right: 0;
&-canvas,
&-cut-canvas {
left: auto;
right: 0;
}
&-toolbar {
left: auto;
right: 0;
}
&-highlight {
left: auto;
right: 0;
.highlight {
left: auto;
right: 50%;
transform: translate(50%, -50%);
}
}
}
}
.canvans-btn {
font-size: 13px;
color: #fff;
font-weight: 400;
display: flex;
justify-content: center;
align-items: center;
cursor: pointer;
}
index.tsx code
import { AvatarCropper } from './avatarcropper'
export type {
AvatarCropperProps,
AvatarCropperToolbarPosition,
AvatarCropperSizeType,
AvatarCropperSourceType,
AvatarCropperShape
} from './avatarcropper'
export default AvatarCropper
type.ts code
import type { CSSProperties, ReactNode } from 'react'
export interface BasicComponent {
className?: string
style?: CSSProperties
children?: ReactNode
id?: string
}
export const ComponentDefaults = {
className: '',
style: {}
}
use-touch.ts code
import React, { useRef } from 'react'
const MIN_DISTANCE = 10
type Direction = '' | 'vertical' | 'horizontal'
function getDirection(x: number, y: number) {
if (x > y && x > MIN_DISTANCE) {
return 'horizontal'
}
if (y > x && y > MIN_DISTANCE) {
return 'vertical'
}
return ''
}
export function useTouch() {
const startX = useRef(0)
const startY = useRef(0)
const deltaX = useRef(0)
const deltaY = useRef(0)
const delta = useRef(0)
const offsetX = useRef(0)
const offsetY = useRef(0)
const direction = useRef<Direction>('')
const last = useRef(false)
const velocity = useRef(0)
const touchTime = useRef<number>(Date.now())
const isVertical = () => direction.current === 'vertical'
const isHorizontal = () => direction.current === 'horizontal'
const reset = () => {
touchTime.current = Date.now()
deltaX.current = 0
deltaY.current = 0
offsetX.current = 0
offsetY.current = 0
delta.current = 0
direction.current = ''
last.current = false
}
const start = (event: React.TouchEvent<HTMLElement>) => {
reset()
touchTime.current = Date.now()
startX.current = event.touches[0].clientX
startY.current = event.touches[0].clientY
}
const move = (event: React.TouchEvent<HTMLElement>) => {
const touch = event.touches[0]
// Fix: Safari back will set clientX to negative number
deltaX.current = touch.clientX < 0 ? 0 : touch.clientX - startX.current
deltaY.current = touch.clientY - startY.current
offsetX.current = Math.abs(deltaX.current)
offsetY.current = Math.abs(deltaY.current)
delta.current = isVertical() ? deltaY.current : deltaX.current
if (!direction.current) {
direction.current = getDirection(offsetX.current, offsetY.current)
}
}
const end = (event: React.TouchEvent<HTMLElement>) => {
last.current = true
velocity.current = Math.sqrt(deltaX.current ** 2 + deltaY.current ** 2) / (Date.now() - touchTime.current)
}
return {
end,
move,
start,
reset,
touchTime,
startX,
startY,
deltaX,
deltaY,
delta,
offsetX,
offsetY,
direction,
isVertical,
isHorizontal,
last
}
}
utils.ts code
export const isObject = (val: unknown): val is Record<any, any> => val !== null && typeof val === 'object'
// eslint-disable-next-line @typescript-eslint/ban-types
export const isFunction = (val: unknown): val is Function => typeof val === 'function'
export const isPromise = <T = any>(val: unknown): val is Promise<T> => {
return isObject(val) && isFunction(val.then) && isFunction(val.catch)
}
export const upperCaseFirst = (str: string) => {
str = str.toLowerCase()
str = str.replace(/\b\w+\b/g, (word) => word.substring(0, 1).toUpperCase() + word.substring(1))
return str
}
export const clamp = (num: number, min: number, max: number): number => Math.min(Math.max(num, min), max)
export function preventDefault(event: React.TouchEvent<HTMLElement> | TouchEvent, isStopPropagation?: boolean) {
if (typeof event.cancelable !== 'boolean' || event.cancelable) {
event.preventDefault()
}
if (isStopPropagation) {
event.stopPropagation()
}
}
简单实例
<AvatarCropper shape="round" onConfirm={cutImage}>
<View className={styles.headImgCenter}>
{!!headImg && <Image className={styles.headImg} src={headImg ?? ''} mode="aspectFill" />}
</View>
</AvatarCropper>
TODO
相关核心逻辑
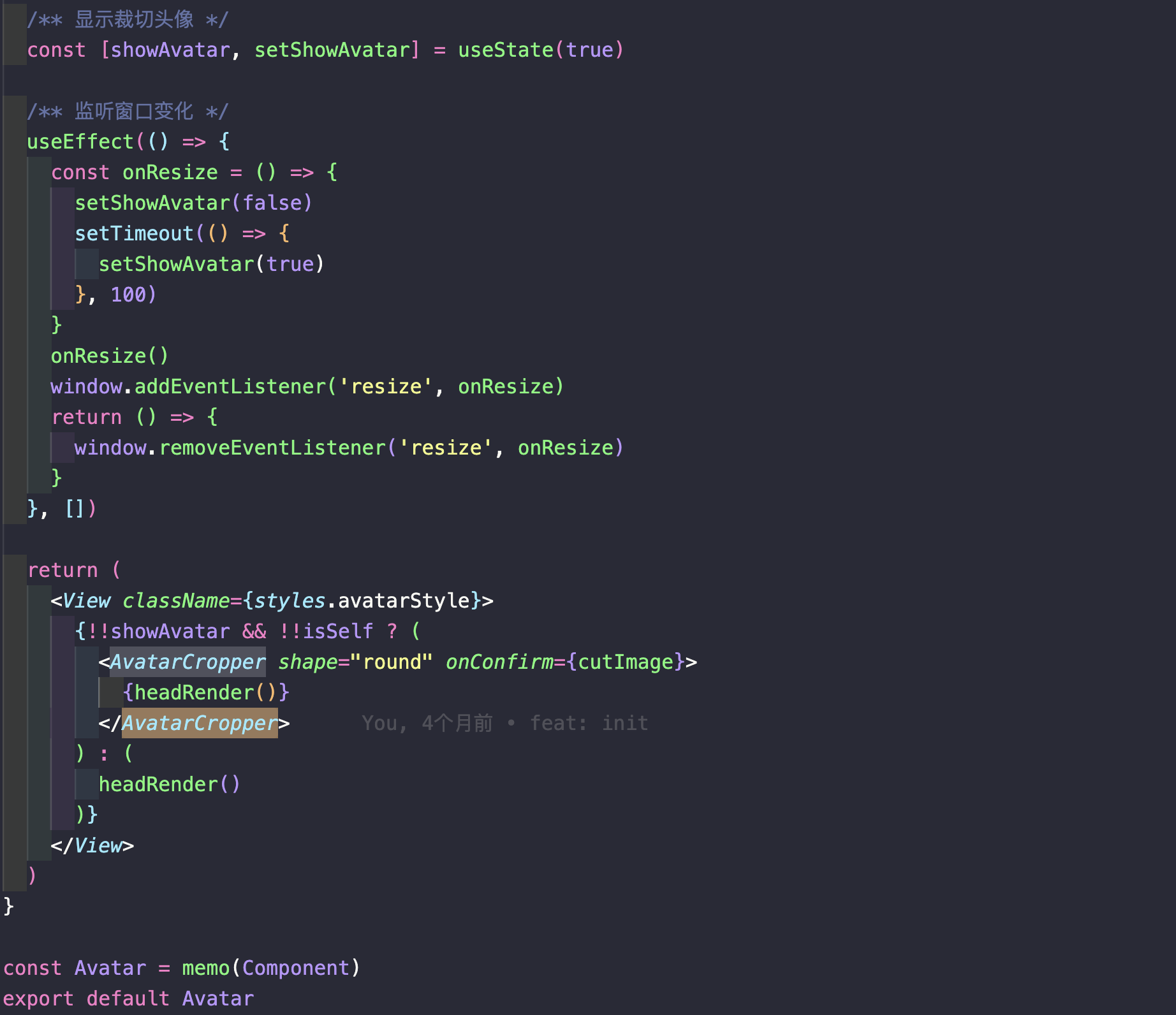